Generate Fingerprint For AuthorizeNet using Java - Surekha Technologies
Generate Fingerprint For AuthorizeNet using Java
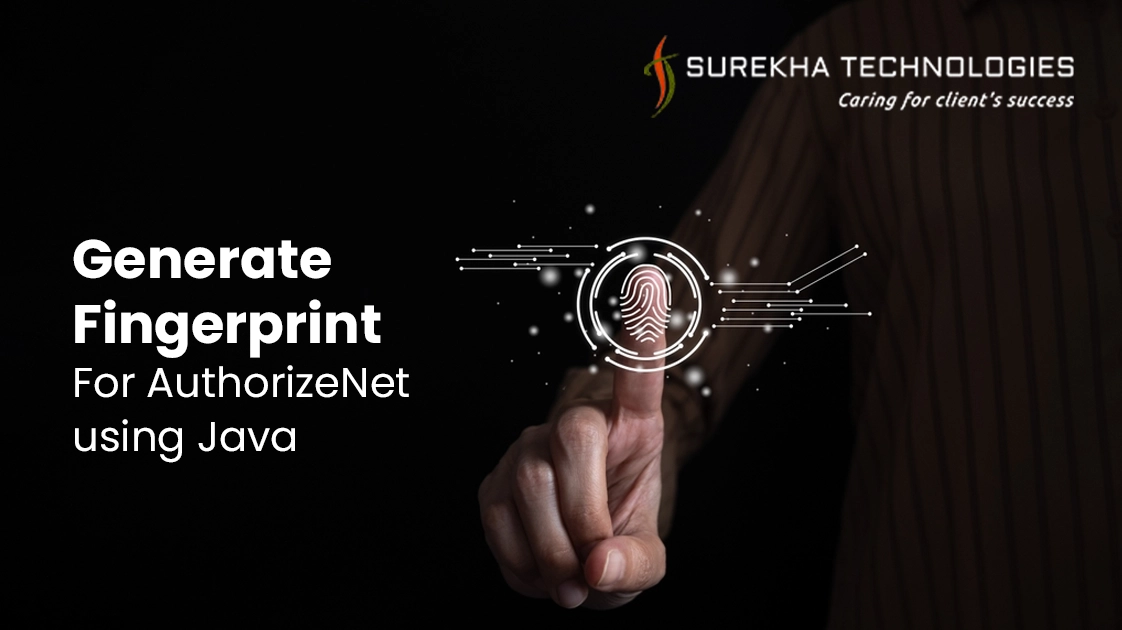
While using Apple Pay and Authorize.Net to process payments in iOS mobile app if you have chosen SDK method for communication between Apple pay and Authorize.Net, you need to generate fingerprint for each transaction.
Refer this for more information : http://www.authorize.net/support/ApplePay_Getting_Started.pdf
According to documentation of Authorize.Net for fingerprint : -
The HMAC-MD5 algorithm is used only for generating the unique transaction fingerprint. The transaction fingerprint must be generated for each transaction by a server-side script on the merchant’s web server and inserted into the transaction request. The payment gateway uses the same mutually exclusive merchant information to decrypt the transaction fingerprint and authenticate the transaction.
Here I am going to show you how to generate fingerprint using HMAC-MD5 algorithm in java.
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.util.Random;
import javax.crypto.Mac;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import org.apache.commons.codec.binary.Hex;
import org.apache.log4j.Logger;
public class GenerateFingerprint {
private static final Logger logger = Logger.getLogger(GenerateFingerprint.class);
public static void main(String[] args) {
// provided by authorize.net
String transactionKey = "{transactionkey}";
// provided by authorize.net
String apiLoginId = "{apiloginid}";
String amount = "20";
// currency Code
String currencyCode = "USD";
try {
// generate timestamp in sequence number
long timeStamp = System.currentTimeMillis() / 1000;
// generate random sequence number
Random random = new Random();
long loginSequence = random.nextInt(100000000);
// section use java Cryptography functions to generate a fingerprint
// convert transaction key to Secret key object
SecretKey key = new SecretKeySpec(transactionKey.getBytes(), "HmacMD5");
// create a MAC object to generate the fingerprint using the HmacMD5 algorithm
Mac mac = Mac.getInstance("HmacMD5");
mac.init(key);
// add caret(^) between input values as per documented by authorizenet
String inputString = apiLoginId + "^" + loginSequence + "^" + timeStamp + "^" + amount + "^" + currencyCode;
// get String as bytes
byte[] inputBytes= inputString.getBytes();
// create digest from byte array
byte[] result = mac.doFinal(inputBytes);
// convert HMAC byte array to char array.
char[] resultCharArray = Hex.encodeHex(result);
// convert char array to string
String fingerprint = new String(resultCharArray);
logger.info("Login Sequence :: " + loginSequence);
logger.info("TimeStamp :: " + timeStamp);
logger.info("-----------------------------------------------");
logger.info("Fingerprint :: " + fingerprint);
logger.info("-----------------------------------------------");
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
} catch (InvalidKeyException e) {
e.printStackTrace();
}
}
}
To run above program you need apache commons codec jar for converting HMAC byte array to character array. You can find it from here :
https://commons.apache.org/proper/commons-codec/
You can also check whether generated fingerprint is correct or not from Response Code 99 Tool by giving required values like transaction key, timestamp etc.
For professional paid support, you may contact us at [email protected] .
For Your Business Requirements