A callout is the view that pops up when tapping on an annotation view. By default, iOS map callout components are good enough for very limited customization. If you need more customization here are the simple steps to create custom callout on MKMapView.
Steps :
Create custom annotation class
Create custom callout view(xib)
Create a swift CustomCalloutView class which extends UIView.
Create a swift CustomMKPinAnnotationView Class which extends MKPinAnnotationView
Create MapViewController(UIViewConteroller) which extends MKMapViewDelegate
Let’s go into details.
-
1. Create custom annotation class
iOS map annotations are described not as a class, but as Protocol. You need to conform to the MKAnnotation protocol. An object that adopts this protocol must implement the coordinate property. The other methods of this protocol are optional.
class CustomAnnotation: NSObject, MKAnnotation {
let placeName: String?
let placeLocationName: String?
let description: String?
let coordinate: CLLocationCoordinate2D
init(placeName: String, placeLocationName: String, description:String, coordinate: CLLocationCoordinate2D) {
self.placeName = placeName
self.placeLocationName = placeLocationName
self.description = description
self.coordinate = coordinate
super.init()
}
}
-
2. Create custom callout view
Create xib file for custom callout(new file -> User Interface -> view) and design it as per your needs.
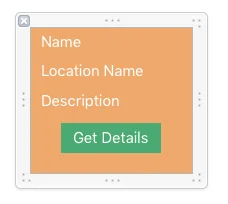
-
3. Create a swift CustomCalloutView class which extends UIView.
Override hitTest and pointInside in your callout view. Create outlet of labels from callout and write action method of button.
-
4. Create a swift CustomMKPinAnnotationView Class which extends MKPinAnnotationView
Here you will assign values to custom callout’s labels in setSelected method.
class CustomMKPinAnnotationView: MKPinAnnotationView {
override func setSelected(selected: Bool, animated: Bool) {
//Set text of labels in custom callout
}
override func hitTest(point: CGPoint, withEvent event: UIEvent?) -> UIView? {
…
…
}
}
-
5. Create MapViewController(UIViewConteroller) which extends MKMapViewDelegate
You need to add your custom annotations in iOS map from viewDidLoad method and viewForAnnotation delegate method is the one that ensures that the annotation's pin is of class CustomMKPinAnnotationView.
private let reuseIdentifier = "CustomPin"
class ViewController: UIViewController, MKMapViewDelegate {
@IBOutlet weak var mapView: MKMapView!
override func viewDidLoad() {
super.viewDidLoad()
//Add annotations
let customAnnotation = CustomAnnotation(name: "Surekhatech", locationName: "India", shortDescription: "One stop solution", coordinate: CLLocationCoordinate2D(latitude: latitude, longitude: longitude))
self.mapView.addAnnotation(customAnnotation)
self.mapView.showAnnotations([customAnnotation], animated: true)
}
//viewForAnnotation delegate method is the one that ensures that the annotation's pin
// is of class CustomMKPinAnnotationView.
func mapView(mapView: MKMapView!, viewForAnnotation annotation: MKAnnotation!) -> MKAnnotationView! {
let pin = mapView.dequeueReusableAnnotationViewWithIdentifier(reuseIdentifier) ?? CustomMKPinAnnotationView(annotation: annotation, reuseIdentifier:reuseIdentifier)!
pin.canShowCallout = false
return pin
}
…
…
}
That’s it. Your app is reday to run.
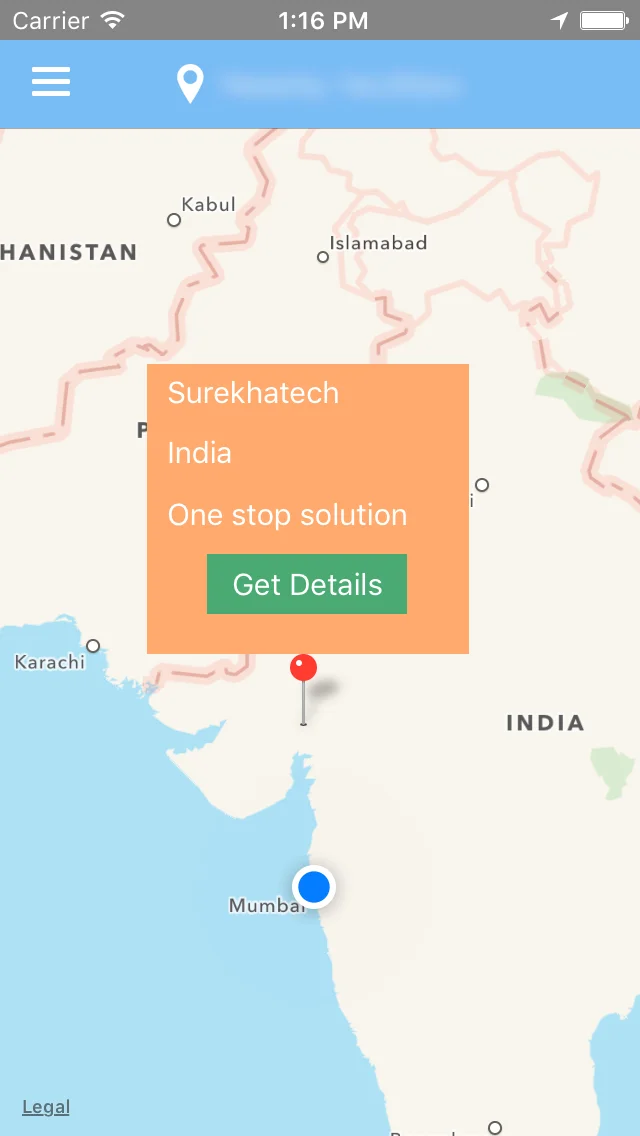