Liferay provides support for Inter-Portlet Communication (IPC) to enable seamless interaction between multiple portlets, making it especially valuable for use cases such as user dashboards or workflows that require real-time communication across portlets.
What Is Inter-Portlet Communication (IPC)?
Inter-Portlet Communication in Liferay is how one portlet can send data or notification to another portlet on the same page or another page. in simple words we can say it is Liferay Portlet Communication. IPC allows for real-time updates, message passing, and rendering of dynamic content without the overhead of loading the entire page.
Why IPC Matters in Liferay Portals?
Liferay portlets are built to be modular and reusable, working independently. But in many cases, they need to interact with each other to achieve specific use cases. Some examples include:
- Refreshing charts when a filter value is changed in another portlet.
- A personalized message or alert being shown across different portlets.
- Refreshing charts when a filter value is changed in another portlet.Real-time use of collaboration tools within a dashboard, forms, etc.
IPC greatly expands usability and modular reusability while keeping the code base organized and clean.
There are four ways to make inter portlet communication:
- Using Portlet Session
- Using Public Render Parameters
- Using Events
- Using AJAX
In this blog, we'll focus on two IPC methods:
- Session-based IPC
- AJAX-based IPC
Prerequisites for Implementing IPC in Liferay
Before you begin, make sure you have the following:
- A working Liferay DXP or CE setup
- Using Public Render Two or more custom portlets developed using MVC
- Basic knowledge of Java, JSP, and JavaScript
- Familiarity with AJAX and session management in Java web applications
Project Setup: Creating Sender and Receiver Portlets
Sender Portlet – collects input and shares it
Receiver Portlet – listens for data and displays it
You can use either the Liferay Blade CLI or Liferay Dev Studio to create both modules.
Note: Make sure both portlets are deployed on the same page in your site for IPC to function properly.
Session-Based Inter-Portlet Communication:
Step 1: Add properties in portal files:
Both portlets require specific Propeties in their default portlet class files:
com.liferay.portlet.private-session-attributes=false
javax.portlet.version=3.0
These ensure that session attributes are accessible across portlets.
Step 2: Sender Portlet – Save Message to Session
In your MVC Action Command:
@Override
protected void doProcessAction(ActionRequest actionRequest, ActionResponse actionResponse) throws Exception {
String message = ParamUtil.getString(actionRequest, "messageSession");
PortletSession session = actionRequest.getPortletSession();
session.setAttribute(SenderWebPortletKeys.KEY_MESSAGE, message, PortletSession.APPLICATION_SCOPE);
}
This saves the message into the shared session under the application scope.
Step 3: Receiver Portlet – Read from Session
In your doView method of the Receiver Portlet:
@Override
public void doView(RenderRequest renderRequest, RenderResponse renderResponse)
throws IOException, PortletException {
PortletSession session = renderRequest.getPortletSession();
String userMessage = (String) session.getAttribute(ReceiverWebPortletKeys.KEY_MESSAGE, PortletSession.APPLICATION_SCOPE);
renderRequest.setAttribute(ReceiverWebPortletKeys.KEY_USER_MESSAGE, userMessage);
super.doView(renderRequest, renderResponse);
}
This code retrieves the session value and sets it for use in the JSP.
Step 4: Add constants and Language Property from sender side:
SenderWebPortletKeys.java:
public static final String KEY_MESSAGE = "message";
public static final String MESSAGE_PASS_EVENT = "messagePassEvent";
public static final String MESSAGE_PARAM = "testParam";
From Sender Language Property File:
message=Enter message
messagePassEvent=messagePassEvent
testParam=Test parameter
Step 5: Add constants and Language Property from receiver side:
ReceiverWebPortletKeys.java:
public static final String KEY_MESSAGE = "message";
public static final String KEY_USER_MESSAGE = "userMessage";
public static final String CLIENT_MESSAGE_ID = "clientMessage";
public static final String CLIENT_MESSAGE_EVENT = "messagePassEvent";
public static final String CLIENT_MESSAGE_PARAM = "testParam";
Receiver Language Property File:
clientMessage=Displaying received value using session
clientMessageId=AJAX IPC
messagePassEvent=messagePassEvent
testParam=Test parameter
AJAX-Based Inter-Portlet Communication:
The AJAX Inter-Portlet Communication (IPC) in Liferay allows portlets to communicate with each other asynchronously without a full-page refresh. The method uses client-side JavaScript (usually AUI or jQuery) to send and retrieve data between portlets.
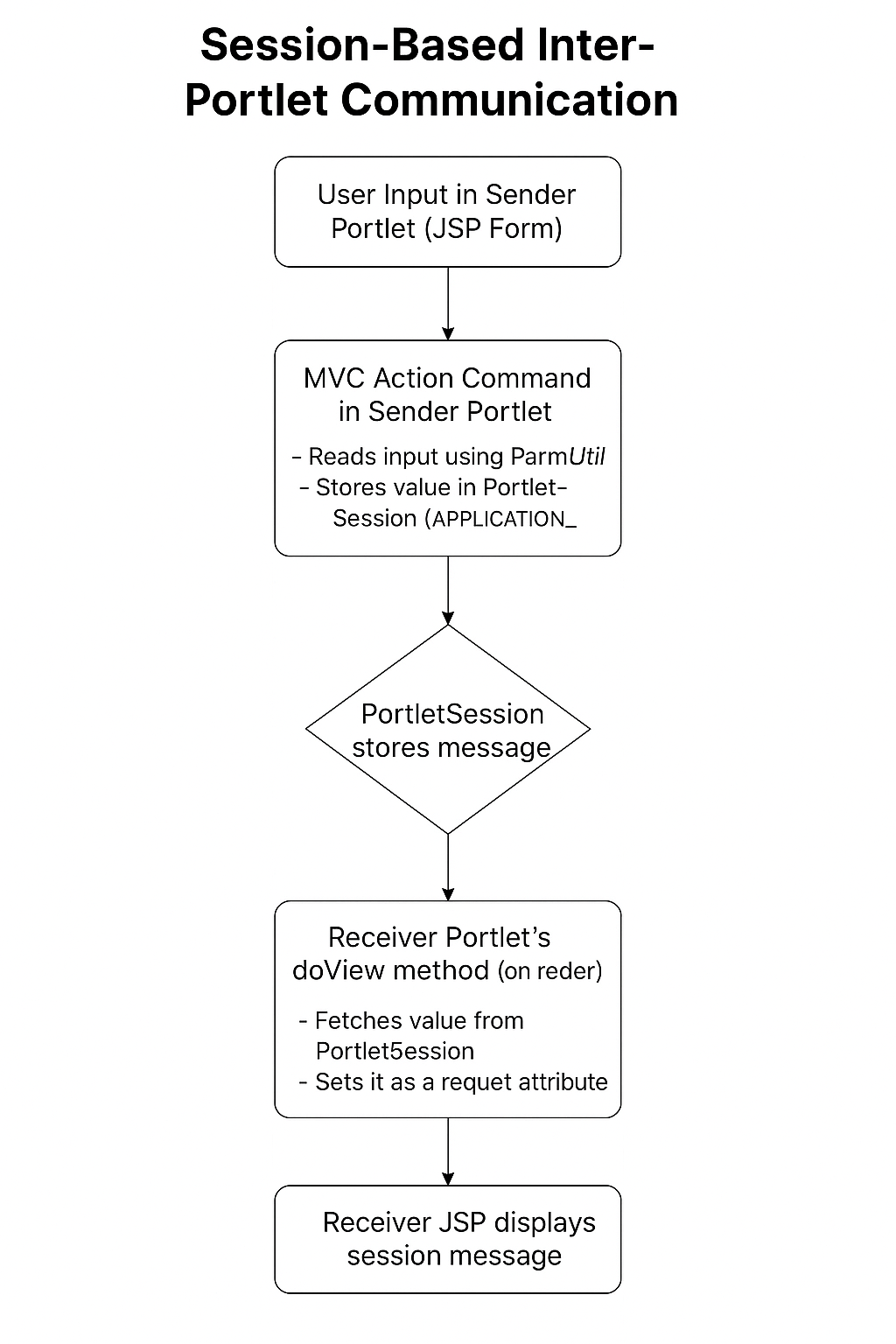
Sender Portlet JSP Snippet
<input type="text" id="message" name="message" placeholder="Enter message" />
<input type="button" value="Send" onClick="sendMessage()" />
<aui:script>
function sendMessage() {
const messageEvent = document.getElementById('message').value;
Liferay.fire('<%= SenderWebPortletKeys.MESSAGE_PASS_EVENT %>', {
<%= SenderWebPortletKeys.MESSAGE_PARAM %>: "Displaying received value using ajax: " + messageEvent
});
}
</aui:script>
Here, a JavaScript event is triggered using Liferay.fire, passing a custom message.
Receiver Portlet JSP Snippet
<p id="<%= ReceiverWebPortletKeys.CLIENT_MESSAGE_ID %>"></p>
<script>
Liferay.on('<%= ReceiverWebPortletKeys.CLIENT_MESSAGE_EVENT %>', function(event) {
const userMessage = event.<%= ReceiverWebPortletKeys.CLIENT_MESSAGE_PARAM %>;
document.getElementById('<%= ReceiverWebPortletKeys.CLIENT_MESSAGE_ID %>').innerHTML = userMessage;
});
</script>
The Liferay.on() function listens for the custom event and updates the content dynamically.
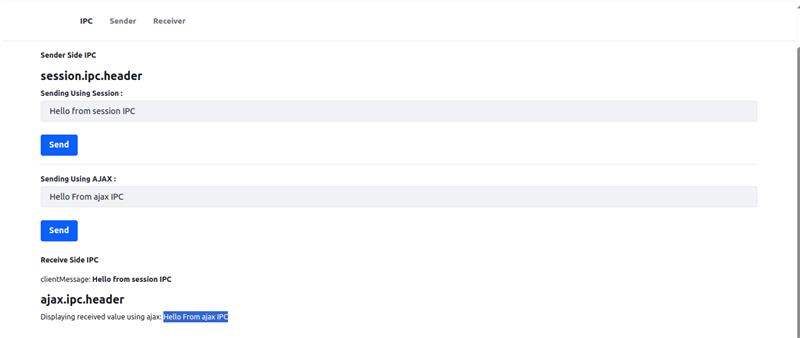
Use Case: Session-Based IPC vs. Client-Side AJAX IPC
Session-based IPC |
AJAX-based IPC |
Liferay's session-based IPC allows sharing data between portlets across different pages by storing values in the shared session scope. |
AJAX-based IPC is only possible if the sender and receiver portlets are on the same page. |
It's perfect for passing information such as user inputs or selections (e.g., from one portlet on Page A to another portlet on Page B). |
It enables real-time, seamless communication using JavaScript events without a page reload. |
Session IPC is a one-way communication method for transferring data. |
Synchronization is instant and based on client-side interaction. |
Typical Issues and Troubleshooting:
here are a few common scenarios developers experience with IPC:
- Session value is null in the receiver: Verify private-session-attributes=false.
- Version mismatch error: Ensure javax.portlet.version=3.0 is set.
- JavaScript did not receive event: Verify that name and keys are the same in both sender and receiver.
Conclusion
Implementing Inter-Portlet Communication (IPC) in Liferay using Session-based and AJAX-based methods unlocks powerful capabilities for creating dynamic, interactive user interfaces. These communication techniques enhance interoperability between components, allowing for seamless data sharing and real-time interactions across portlets.
Whether you're building dashboards, business forms, or real-time collaboration tools, mastering IPC gives you the flexibility to deliver exceptional user experiences. Session-based IPC offers a reliable, backend-driven approach, while AJAX-based IPC enables responsive, client-side communication—together covering a wide range of use cases.
At Surekha Technologies, our Liferay Development Services are designed to help you implement these advanced features with ease, ensuring your portal solutions are scalable, efficient, and user centric.